Solutions to the Practice Questions#
Hierarchies for Inheritance#
The following code goes in the
Rectangle
class:public double calcDiagonalLength(){ return Math.sqrt(width * width + length * length); }//calcDiagonalLength
In
main
method:Square aSquare = new Square(8); System.out.println("Length of diagonal: " + aSquare.calcDiagonalLength());
In
main
method:Rectangle aRect = new Rectangle(5, 3); System.out.println("Length of diagonal: " + aRect.calcDiagonalLength());
Setters and Getters#
In
Rectangle
class:public void setWidth(double width){ this.width = width; }
In
Square
class:public void setWidth(double width){ this.width = width; this.length = width; }
Default Constructor#
In class
Rectangle
:public Rectangle(){ this(1, 2); }
In class
RightTriangle
:public RightTriangle(){ this(2, 5); }
In class
Circle
:public Circle(){ this(1); }
A Representation to Print#
In class
Circle
:@Override public String toString(){ return getClass().getName() + " radius = " + radius; }
In class
RightTriangle
:@Override public String toString(){ return getClass().getName() + " base = " + base + " height = " + height; }
No code to write as
toString()
will be correctly inherited from theRectangle
class.
Every Class Extends Object#
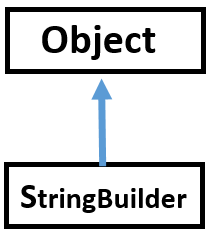
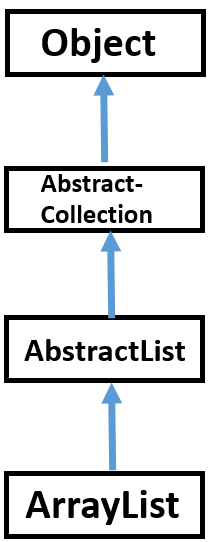
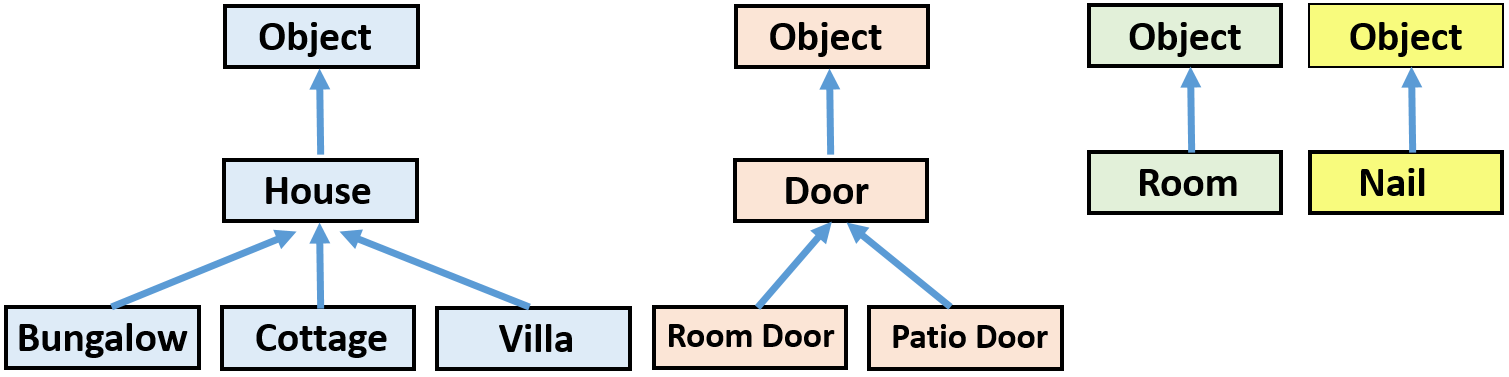
[Back to Questions](chapter3:extendsObject:practice)