Visualize Your Program#
Every Java statement does one particular, non-ambiguous thing, should be written on one line, and usually ends in a semicolon. A Java program is a sequence of Java statements. In order to write computer programs, you should be able to execute them yourself, in your head, with pencil and paper, or writing on a tablet. You need to have a picture of what is going on, but this picture does not need to be a complete model of a computer. Every computer program manipulates memory; input and output (I/O) are optional. Our visualization will contain an idea of what memory might look like, e.g. a bunch of boxes, and that a program may take input and produce output. This model is a simplification of the von Neumann architecture you will study in future years.
Example Visualization#
Given this Java program:
int x = 4;
x = x + x;
System.out.println("x is: " + x);
x is: 8
Here is a simple illustration of its execution:
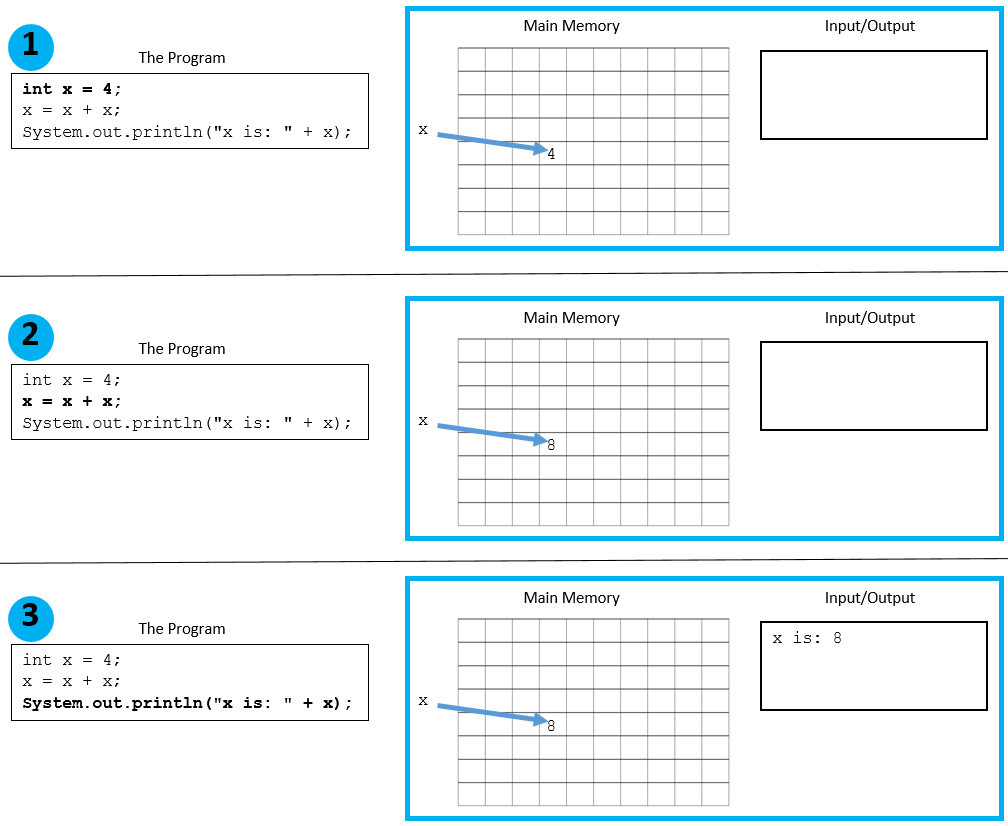
Each statement, shown in bold, causes something to happen in memory or in the Input/Output window.
In the model, we saw that memory is a grid of cells where each cell holds one value. Different data types may require a different number of memory cells, which you will learn about in a first course on computer architecture. Since this is not as important when working in Java, it is sufficient to simply think about things being in memory in a “big enough” spot, and overwritten when (re-)assignment occurs. We might then draw our program as:
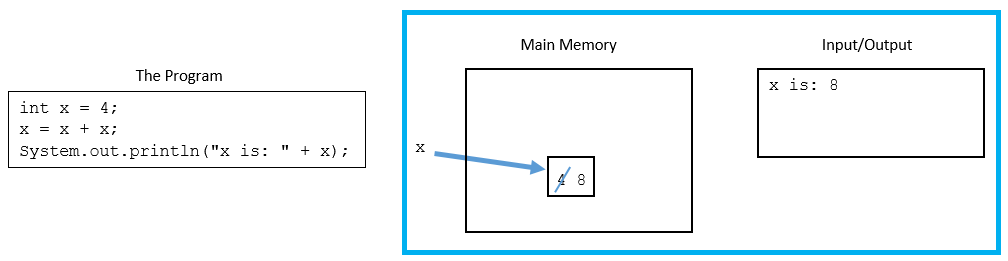
An important realization is that the name you have given to a memory location, i.e. the variable name, exists only outside of the run-time or execution time of your program. In other words, those names are only useful to people when writing or reading a program. Those names will be converted into binary as addresses in memory so that the computer knows where to find our data at run-time.
Without Running the Program Know What Will Happen#
As we program more, these pictures will naturally be “in our heads”, and we won’t need to explicitly draw them. When people are learning to program, they often do what I call “programming by guessing.” By this, I mean entering some code, running the programming, and then changing values almost at random in the code or changing the order of lines in the code, all without thinking about what is going on in the computer. Our computers are so fast, that this seems like a legitimate way to program (make a change, run, error, repeat) and this technique might produce a “working” program on small problems. However, this technique is not helpful in real-world problems. You must understand how your program executes. You must be able to be “the computer.”
Stop Programming By Guessing
We always start simple, and perhaps programming by guessing “works” but it doesn’t allow you to learn to program. You should be able to execute every program in your head, and be able to draw what happens.
Practice Questions#
Draw a memory diagram for the following Java program:
int jasper; int banff; banff = 45; jasper = banff % 8; jasper = jasper / 3;
Draw a memory diagram for the following Java program:
double jade; int emerald; int ruby; jade = 76.0 / 5; emerald = 76 / 5;