Every Class Extends Object#
If a new class is created in Java, but the extends
reserved word is not used, a hierarchy is created nevertheless. Every class inherits from the built-in, predefined class called Object
. This means that the hierarchies for the shapes we have been building in the previous sections are really:
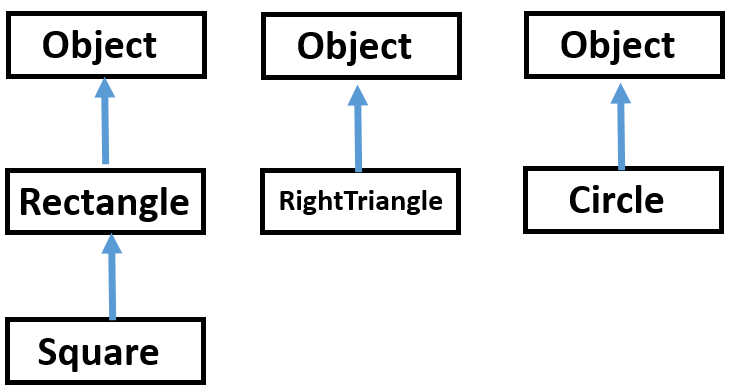
The Object
class has a toString()
method implemented within it and this toString()
method gives the class name and the memory location. When we write our own toString()
method, we are always blocking the inheritance of the built-in toString()
and so we add the “@override” annotation to all toString()
s.
Arrays Are Also Objects#
Any array data type used in a Java program also inherits from the Object
data type. For example, if we create a 1d array of int
s and a 2d array of char
s, we will have these hierarchies:
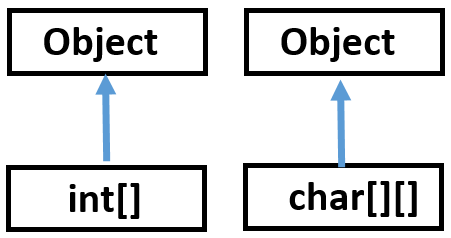
A String is an Object#
You guessed it - String
s are also Object
s.
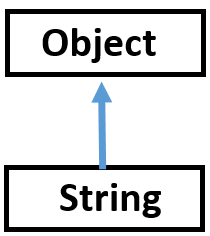
This can be determined by looking at the Java documentation for String.
new Makes or Creates an Object in Memory#
Any time that the new
reserved word is used, an object is created. This object becomes alive at run-time when the new
command is executed. The object lives in memory until the closing bracket is reached to close the block in which the object is declared. Objects are also called instances.
Practice Questions#
Draw the hierarchy diagram for a
StringBuilder
. Java documentation for StringBuilder.Draw the hierarchy diagram for an
ArrayList
(this is a new concept). Java documentation for ArrayList.Suppose you are writing Java code about the following: house, bungalow, room, door, cottage, room door, patio door, villa, and nail. What would be a good hierarchy diagram?